
What We Do
- Our Focus is You
We focus on your online needs so that you can focus on your customer's needs. There are numerous way in which we can help you. We begin by reviewing your business goals, your marketing objectives, and your competitive position, and then we develop your online presence to meet your needs. - Dynamic Content
Today's web applications require dynamically driven content, and much of that content is provided by a database and a programming language operating in harmony to produce the desired results. MySQL and PHP are two of the most popular web development tools utilized to produce dynamic web sites. We can help you take control and begin building truly dynamic web content which is easier to maintain, is more responsive to your users, and can alter its appearance based upon differing situations. Contact Us today to discuss your custom development needs. - Content Management Systems (CMS)
We can deploy CMS applications to allow organizations to edit content easily, which significantly cuts down on costly professional maintenance fees. Our CMS expertise covers Joomla, Wordpress and Blogger platforms. We are ready to help you choose which works best for your specific needs. Contact Us today to begin taking control of your content.
Powered by Blogger.
Methods: A Deeper Look
Methods
A method is a group of statements that together perform a task. Every C# program has at least one class with a method named Main.
To use a method, you need to:
- Define the method
- Call the method
Defining Methods in C#:
When you define a method, you basically declare the elements of its structure. The syntax for defining a method in C# is as follows:
<Access Specifier> <Return Type> <Method Name>(Parameter List) { Method Body }
- The best way to develop and maintain a large app is to construct it from small, simple pieces.
- Common ways of packaging code are properties, methods, classes and namespaces.
- The Framework Class Library provides many predefined classes that contain methods for performing common tasks.
Modularizing Programs
Methods allow you to modularize an app by separating its tasks into reusable units. Methods that you write are sometimes referred to as user-defined methods. The “divide-and-conquer” approach makes app development more manageable by constructing apps from small, simple pieces. Software reusability is utilizing existing methods to build blocks to create new apps.
Following are the various elements of a method:
- Access Specifier: This determines the visibility of a variable or a method from another class.
- Return type: A method may return a value. The return type is the data type of the value the method returns. If the method is not returning any values, then the return type is void.
- Method name: Method name is a unique identifier and it is case sensitive. It cannot be same as any other identifier declared in the class.
- Parameter list: Enclosed between parentheses, the parameters are used to pass and receive data from a method. The parameter list refers to the type, order, and number of the parameters of a method. Parameters are optional; that is, a method may contain no parameters.
- Method body: This contains the set of instructions needed to complete the required activity.
Example:
Following code snippet shows a function FindMax that takes two integer values and returns the larger of the two. It has public access specifier, so it can be accessed from outside the class using an instance of the class.
class NumberManipulator { public int FindMax(int num1, int num2) { /* local variable declaration */ int result; if (num1 > num2) result = num1; else result = num2; return result; } ... }
Calling Methods
The code that calls a method is known as the client code—that is, any statement that calls the object’s method from outside the object is a client of the method. An analogy to the method-call-and-return structure is the hierarchical form of management (Figure 7.1).
The boss method does not know how the worker method performs its designated tasks. The worker may also call other worker methods. This hiding of implementation details promotes good software engineering.
You can call a method using the name of the method. The following example illustrates this:
using System; namespace CalculatorApplication { class NumberManipulator { public int FindMax(int num1, int num2) { /* local variable declaration */ int result; if (num1 > num2) result = num1; else result = num2; return result; } static void Main(string[] args) { /* local variable definition */ int a = 100; int b = 200; int ret; NumberManipulator n = new NumberManipulator(); //calling the FindMax method ret = n.FindMax(a, b); Console.WriteLine("Max value is : {0}", ret ); Console.ReadLine(); } }
When the above code is compiled and executed, it produces the following result:
Max value is : 200
You can also call public method from other classes by using the instance of the class. For example, the method FindMax belongs to the NumberManipulator class, you can call it from another class Test.
using System; namespace CalculatorApplication { class NumberManipulator { public int FindMax(int num1, int num2) { /* local variable declaration */ int result; if (num1 > num2) result = num1; else result = num2; return result; } } class Test { static void Main(string[] args) { /* local variable definition */ int a = 100; int b = 200; int ret; NumberManipulator n = new NumberManipulator(); //calling the FindMax method ret = n.FindMax(a, b); Console.WriteLine("Max value is : {0}", ret ); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
Max value is : 200
Recursive Method Call
A method can call itself. This is known as recursion. Following is an example that calculates factorial for a given number using a recursive function:
using System; namespace CalculatorApplication { class NumberManipulator { public int factorial(int num) { /* local variable declaration */ int result; if (num == 1) { return 1; } else { result = factorial(num - 1) * num; return result; } } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); //calling the factorial method Console.WriteLine("Factorial of 6 is : {0}", n.factorial(6)); Console.WriteLine("Factorial of 7 is : {0}", n.factorial(7)); Console.WriteLine("Factorial of 8 is : {0}", n.factorial(8)); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
Factorial of 6 is: 720 Factorial of 7 is: 5040 Factorial of 8 is: 40320
Passing Parameters to a Method
When method with parameters is called, you need to pass the parameters to the method. In C#, there are three ways that parameters can be passed to a method:
Mechanism | Description |
---|---|
Value parameters | This method copies the actual value of an argument into the formal parameter of the function. In this case, changes made to the parameter inside the function have no effect on the argument. |
Reference parameters | This method copies the reference to the memory location of an argument into the formal parameter. This means that changes made to the parameter affect the argument. |
Output parameters | TThis method helps in returning more than one value. |
Value Parameters
This is the default mechanism for passing parameters to a method. In this mechanism, when a method is called, a new storage location is created for each value parameter.
The values of the actual parameters are copied into them. So, the changes made to the parameter inside the method have no effect on the argument. The following example demonstrates the concept:
using System; namespace CalculatorApplication { class NumberManipulator { public void swap(int x, int y) { int temp; temp = x; /* save the value of x */ x = y; /* put y into x */ y = temp; /* put temp into y */ } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a = 100; int b = 200; Console.WriteLine("Before swap, value of a : {0}", a); Console.WriteLine("Before swap, value of b : {0}", b); /* calling a function to swap the values */ n.swap(a, b); Console.WriteLine("After swap, value of a : {0}", a); Console.WriteLine("After swap, value of b : {0}", b); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
Before swap, value of a :100 Before swap, value of b :200 After swap, value of a :100 After swap, value of b :200
Reference Parameters
A reference parameter is a reference to a memory location of a variable. When you pass parameters by reference, unlike value parameters, a new storage location is not created for these parameters. The reference parameters represent the same memory location as the actual parameters that are supplied to the method.
In C#, you declare the reference parameters using the ref keyword. The following example demonstrates this:
using System; namespace CalculatorApplication { class NumberManipulator { public void swap(ref int x, ref int y) { int temp; temp = x; /* save the value of x */ x = y; /* put y into x */ y = temp; /* put temp into y */ } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a = 100; int b = 200; Console.WriteLine("Before swap, value of a : {0}", a); Console.WriteLine("Before swap, value of b : {0}", b); /* calling a function to swap the values */ n.swap(ref a, ref b); Console.WriteLine("After swap, value of a : {0}", a); Console.WriteLine("After swap, value of b : {0}", b); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
Before swap, value of a : 100 Before swap, value of b : 200 After swap, value of a : 200 After swap, value of b : 100
Output Parameters
A return statement can be used for returning only one value from a function. However, using output parameters, you can return two values from a function. Output parameters are like reference parameters, except that they transfer data out of the method rather than into it.
The following example illustrates this:
using System; namespace CalculatorApplication { class NumberManipulator { public void getValue(out int x ) { int temp = 5; x = temp; } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a = 100; Console.WriteLine("Before method call, value of a : {0}", a); /* calling a function to get the value */ n.getValue(out a); Console.WriteLine("After method call, value of a : {0}", a); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
Before method call, value of a : 100 After method call, value of a : 5
The variable supplied for the output parameter need not be assigned a value the method call. Output parameters are particularly useful when you need to return values from a method through the parameters without assigning an initial value to the parameter. Look at the following example, to understand this:
using System; namespace CalculatorApplication { class NumberManipulator { public void getValues(out int x, out int y ) { Console.WriteLine("Enter the first value: "); x = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Enter the second value: "); y = Convert.ToInt32(Console.ReadLine()); } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a , b; /* calling a function to get the values */ n.getValues(out a, out b); Console.WriteLine("After method call, value of a : {0}", a); Console.WriteLine("After method call, value of b : {0}", b); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
Enter the first value: 7 Enter the second value: 8 After method call, value of a : 7 After method call, value of b : 8
C# Control Statements Part 2 (For , While, and Do Loops)
There are situations that require you to execute a block of code several number of times. In general statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
Programming languages provide various control structures that allow for more complicated execution paths.
A loop statement allows us to execute a statement or group of statements multiple times and following is the general from of a loop statement in most of the programming languages:
Loop Architecture

C# provides following types of loop to handle looping requirements. Click the following links to check their detail.
C# provides the following control statements. Click the following links to check their details.
Programming languages provide various control structures that allow for more complicated execution paths.
A loop statement allows us to execute a statement or group of statements multiple times and following is the general from of a loop statement in most of the programming languages:
Loop Architecture

C# provides following types of loop to handle looping requirements. Click the following links to check their detail.
Loop Type | Description |
---|---|
for loop | Executes a sequence of statements multiple times and abbreviates the code that manages the loop variable. |
while loop | Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body. |
do...while loop | Like a while statement, except that it tests the condition at the end of the loop body |
nested loops | You can use one or more loop inside any another while, for or do..while loop. |
For Loop
A for loop is a repetition control structure that allows you to efficiently write a loop that needs to execute a specific number of times.
Syntax:
The syntax of a for loop in C# is:
for ( init; condition; increment ) { statement(s); }
Here is the flow of control in a for loop:
- The init step is executed first, and only once. This step allows you to declare and initialize any loop control variables. You are not required to put a statement here, as long as a semicolon appears.
- Next, the condition is evaluated. If it is true, the body of the loop is executed. If it is false, the body of the loop does not execute and flow of control jumps to the next statement just after the for loop.
- After the body of the for loop executes, the flow of control jumps back up to the increment statement. This statement allows you to update any loop control variables. This statement can be left blank, as long as a semicolon appears after the condition.
- The condition is now evaluated again. If it is true, the loop executes and the process repeats itself (body of loop, then increment step, and then again condition). After the condition becomes false, the for loop terminates.
Flow Diagram:

Example:
using System; namespace Loops { class Program { static void Main(string[] args) { /* for loop execution */ for (int a = 10; a < 20; a = a + 1) { Console.WriteLine("value of a: {0}", a); } Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 15 value of a: 16 value of a: 17 value of a: 18 value of a: 19
While Loop
A while loop statement in C# repeatedly executes a target statement as long as a given condition is true.
Syntax:
The syntax of a while loop in C# is:
while(condition) { statement(s); }
Here, statement(s) may be a single statement or a block of statements. The condition may be any expression, and true is any non-zero value. The loop iterates while the condition is true.
When the condition becomes false, program control passes to the line immediately following the loop.
Flow Diagram:
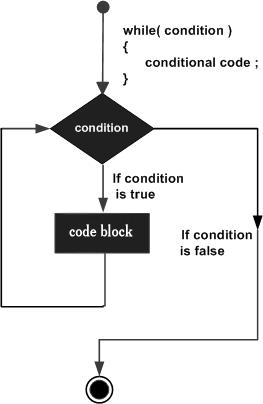
Here, key point of the while loop is that the loop might not ever run. When the condition is tested and the result is false, the loop body will be skipped and the first statement after the while loop will be executed.
Example:
using System; namespace Loops { class Program { static void Main(string[] args) { /* local variable definition */ int a = 10; /* while loop execution */ while (a < 20) { Console.WriteLine("value of a: {0}", a); a++; } Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 15 value of a: 16 value of a: 17 value of a: 18 value of a: 19
Do While Loop
Unlike for and while loops, which test the loop condition at the top of the loop, the do...while loop checks its condition at the bottom of the loop.
A do...while loop is similar to a while loop, except that a do...while loop is guaranteed to execute at least one time.
Syntax:
The syntax of a do...while loop in C# is:
do { statement(s); }while( condition );
Notice that the conditional expression appears at the end of the loop, so the statement(s) in the loop execute once before the condition is tested.
If the condition is true, the flow of control jumps back up to do, and the statement(s) in the loop execute again. This process repeats until the given condition becomes false.
Flow Diagram:
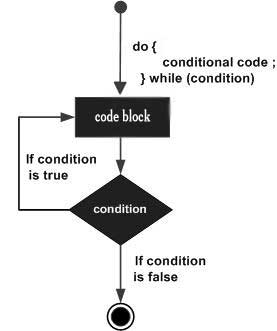
Example:
using System; namespace Loops { class Program { static void Main(string[] args) { /* local variable definition */ int a = 10; /* do loop execution */ do { Console.WriteLine("value of a: {0}", a); a = a + 1; } while (a < 20); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 15 value of a: 16 value of a: 17 value of a: 18 value of a: 19
Nested Loops
C# allows to use one loop inside another loop. Following section shows few examples to illustrate the concept.
Syntax:
The syntax for a nested for loop statement in C# is as follows:
for ( init; condition; increment ) { for ( init; condition; increment ) { statement(s); } statement(s); }
The syntax for a nested while loop statement in C# is as follows:
while(condition) { while(condition) { statement(s); } statement(s); }
The syntax for a nested do...while loop statement in C# is as follows:
do { statement(s); do { statement(s); }while( condition ); }while( condition );
A final note on loop nesting is that you can put any type of loop inside of any other type of loop. For example a for loop can be inside a while loop or vice versa.
Example:
The following program uses a nested for loop to find the prime numbers from 2 to 100:
using System; namespace Loops { class Program { static void Main(string[] args) { /* local variable definition */ int i, j; for (i = 2; i < 100; i++) { for (j = 2; j <= (i / j); j++) if ((i % j) == 0) break; // if factor found, not prime if (j > (i / j)) Console.WriteLine("{0} is prime", i); } Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
2 is prime 3 is prime 5 is prime 7 is prime 11 is prime 13 is prime 17 is prime 19 is prime 23 is prime 29 is prime 31 is prime 37 is prime 41 is prime 43 is prime 47 is prime 53 is prime 59 is prime 61 is prime 67 is prime 71 is prime 73 is prime 79 is prime 83 is prime 89 is prime 97 is prime
Loop Control Statements:
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.C# provides the following control statements. Click the following links to check their details.
Control Statement | Description |
---|---|
break statement | Terminates the loop or switch statement and transfers execution to the statement immediately following the loop or switch. |
continue statement | Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. |
Break
The break statement in C# has following two usage:
- When the break statement is encountered inside a loop, the loop is immediately terminated and program control resumes at the next statement following the loop.
- It can be used to terminate a case in the switch statement.
If you are using nested loops (i.e., one loop inside another loop), the break statement will stop the execution of the innermost loop and start executing the next line of code after the block.
Syntax:
The syntax for a break statement in C# is as follows:
break;
Flow Diagram:
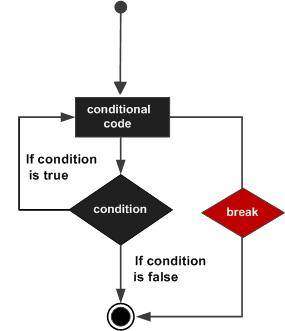
Example:
using System; namespace Loops { class Program { static void Main(string[] args) { /* local variable definition */ int a = 10; /* while loop execution */ while (a < 20) { Console.WriteLine("value of a: {0}", a); a++; if (a > 15) { /* terminate the loop using break statement */ break; } } Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 15
Continue
The continue statement in C# works somewhat like the break statement. Instead of forcing termination, however, continue forces the next iteration of the loop to take place, skipping any code in between.
For the for loop, continue statement causes the conditional test and increment portions of the loop to execute. For the while and do...while loops, continue statement causes the program control passes to the conditional tests.
Syntax:
The syntax for a continue statement in C# is as follows:
continue;
Flow Diagram:

Example:
using System; namespace Loops { class Program { static void Main(string[] args) { /* local variable definition */ int a = 10; /* do loop execution */ do { if (a == 15) { /* skip the iteration */ a = a + 1; continue; } Console.WriteLine("value of a: {0}", a); a++; } while (a < 20); Console.ReadLine(); } } }
When the above code is compiled and executed, it produces the following result:
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 16 value of a: 17 value of a: 18 value of a: 19
The Infinite Loop:
A loop becomes infinite loop if a condition never becomes false. The for loop is traditionally used for this purpose. Since none of the three expressions that form the for loop are required, you can make an endless loop by leaving the conditional expression empty.using System; namespace Loops { class Program { static void Main(string[] args) { for (; ; ) { Console.WriteLine("Hey! I am Trapped"); } } } }When the conditional expression is absent, it is assumed to be true. You may have an initialization and increment expression, but programmers more commonly use the for(;;) construct to signify an infinite loop.
Subscribe to:
Posts
(Atom)